Advanced Programming Techniques
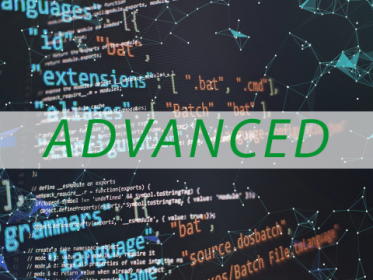
About Course
An Advanced Programming Techniques course is an advanced-level training program designed to teach individuals advanced programming concepts and techniques. This course is typically intended for experienced programmers who already possess a strong foundation in programming fundamentals and want to deepen their skills and understanding of more sophisticated programming concepts.
In an Advanced Programming Techniques course, participants typically learn:
- Data Structures and Algorithms: Advanced data structures (e.g., graphs, trees, hash tables) and algorithms (e.g., sorting, searching, dynamic programming) to solve complex computational problems efficiently.
- Object-Oriented Design Patterns: Advanced design patterns and principles to write scalable, maintainable, and extensible code.
- Concurrency and Multithreading: Techniques to manage multiple tasks concurrently and efficiently utilize system resources in multi-threaded environments.
- Memory Management and Optimization: Strategies for efficient memory allocation and optimization to improve performance and reduce memory overhead.
- Functional Programming: Concepts of functional programming, immutability, and higher-order functions to write concise and expressive code.
- Meta-Programming: Techniques to create code that can modify or generate code dynamically during runtime.
- Software Development Best Practices: Advanced software development methodologies, testing practices, and debugging techniques.
- Software Architecture: Design principles and architectural patterns to develop large-scale and complex software systems.
The Advanced Programming Techniques course challenges participants to solve complex programming problems and equips them with the expertise needed to write efficient, maintainable, and high-performance code. It is valuable for software engineers, developers, and programmers looking to take their programming skills to the next level and work on demanding software projects that require advanced problem-solving and optimization techniques.
Course Content
Advanced Data Structures
-
Advanced data structures such as trees, graphs, and heaps
-
Implementing and working with advanced data structures in various programming languages
-
Use cases for different data structures and their advantages