Java – Programming Fundamentals
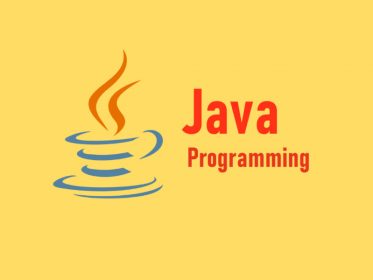
About Course
Java is a widely-used, object-oriented programming language known for its portability, simplicity, and robustness. “Java – Programming Fundamentals” typically refers to a beginner-level course or learning program that covers the foundational concepts of Java programming. These courses are designed to introduce individuals to the basics of Java and help them build a strong foundation for further Java development.
A typical “Java – Programming Fundamentals” course may cover the following key topics:
1. Introduction to Java: Understanding what Java is, its history, and its role in modern software development.
2. Setting Up Java Development Environment: Installing the necessary software (JDK – Java Development Kit, IDE – Integrated Development Environment) to write and run Java code.
3. Basic Syntax: Learning the basic syntax of Java, including data types, variables, operators, and control structures (if-else, loops).
4. Object-Oriented Programming (OOP): Understanding the principles of OOP, such as classes, objects, inheritance, polymorphism, and encapsulation.
5. Methods and Functions: Learning how to define and call methods and functions in Java to organize code and perform specific tasks.
6. Arrays and Collections: Understanding how to work with arrays and Java collections (ArrayList, HashMap, etc.) to store and manipulate data.
7. Exception Handling: Learning how to handle exceptions and errors in Java programs to make them more robust.
8. File I/O: Exploring how to read from and write to files in Java for data storage and retrieval.
9. GUI (Graphical User Interface): An introduction to creating graphical user interfaces using Java’s Swing or JavaFX libraries.
10. Basic Input/Output: Learning how to read input from the console and display output to the console.
11. Introduction to Threads: Understanding the basics of multi-threading in Java to handle concurrent operations.
12. Basic Algorithms and Problem Solving: Applying Java to solve simple programming problems and exercises.
Throughout the course, learners will likely work on various hands-on exercises and projects to practice their Java coding skills and solidify their understanding of the concepts taught.
It’s worth noting that while a “Java – Programming Fundamentals” course provides an excellent starting point, Java is a vast language with many advanced features and applications. As learners progress, they may want to explore more specialized topics, such as web development with Java frameworks (e.g., Spring), Java for Android app development, enterprise-level Java programming, and more.
Additionally, staying updated with the latest versions and enhancements to the Java language and ecosystem is crucial, as Java continues to evolve over time.
Course Content
Introduction to Programming and Java
-
Overview of programming languages and their applications
-
Introduction to Java and its advantages
-
Setting up the Java development environment (JDK, IDE, etc.)